Code refactoring is an essential practice in software development, but it’s often misunderstood or overlooked.
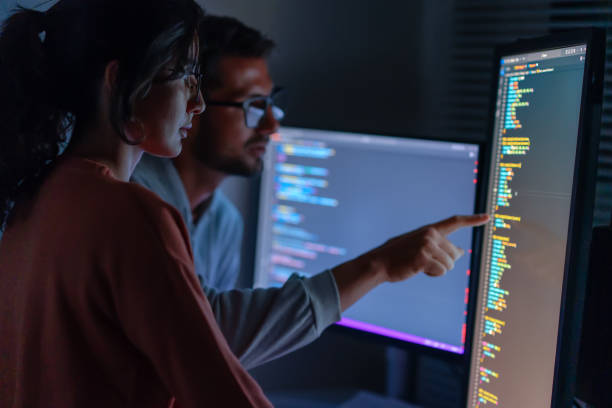
Refactoring refers to the process of restructuring existing code without changing its external behaviour. It is primarily aimed at improving code quality, enhancing maintainability, and making the code easier to understand. In this article, we’ll explore best practices and tips to master the art of code refactoring.
Why refactor code?
Before diving into best practices, let’s first explore why refactoring is necessary:
- Improved readability: Code that is easy to read and understand is easier to maintain and debug. Refactoring helps eliminate complexity and makes code more accessible to team members.
- Easier maintenance: Over time, codebases tend to grow, and minor changes can introduce issues. Refactoring allows for cleaner, more organised code that is easier to modify and extend.
- Reduced technical debt: As projects evolve, it’s easy to accumulate technical debt—poor decisions or shortcuts taken during development. Refactoring helps to reduce this debt and prevent it from growing out of control.
- Better performance: While refactoring is often not done for performance reasons, it can inadvertently lead to performance improvements, especially when simplifying complex logic or removing redundant code.
When should you refactor?
While refactoring is beneficial, it’s important not to do it unnecessarily. Here are some scenarios when refactoring is especially helpful:
- When adding new features: If you’re adding new functionality, check if the existing code is overly complex or hard to work with. Refactor before adding new code to ensure a solid foundation.
- When fixing bugs: If you’re fixing a bug and find the code is difficult to navigate, it might be a good idea to refactor it first, making future debugging easier.
- When revisiting old code: If you’re inheriting an old codebase or revisiting code that hasn’t been touched in a while, refactoring can help modernise the structure and remove outdated patterns.
Best practices for code refactoring
1. Refactor in small increments
Refactoring doesn’t need to be a huge task. In fact, it’s best done in small, incremental steps. Rather than trying to refactor an entire module or function at once, break it down into smaller pieces. This way, you can ensure that each change doesn’t introduce new bugs and that the system still works after each modification.
2. Write tests before refactoring
One of the most important best practices in refactoring is to write tests beforehand, especially unit tests. Writing tests ensures that you can confidently verify the functionality of your code before and after refactoring. If you don’t have sufficient test coverage, you may inadvertently break existing functionality without realising it.
3. Make refactoring a continuous practice
Rather than waiting for large code rewrites, aim to make refactoring an ongoing part of your development workflow. This can involve regularly reviewing code, fixing obvious issues, and refactoring small sections of code as part of regular feature development or bug fixes. By keeping the codebase clean over time, you can avoid the need for massive, disruptive refactoring efforts.
4. Follow the boy scout rule
The Boy Scout Rule states: “Always leave the codebase cleaner than you found it.” If you’re working in a codebase, aim to improve the quality with every change you make. Whether you’re adding a new feature or fixing a bug, look for opportunities to refactor your code and make it more maintainable.
5. Keep it simple
When refactoring, it’s easy to fall into the trap of over-engineering the solution. The goal of refactoring is not to create the most elegant or complex solution, but to make the code easier to maintain and understand. Focus on simplicity and clarity. If a change isn’t necessary or doesn’t provide significant benefits, avoid making it.
6. Use design patterns wisely
Refactoring often involves introducing design patterns to solve common problems. However, it’s important to use patterns judiciously. Design patterns should be introduced when they improve the clarity or structure of the code, but be careful not to introduce unnecessary complexity. Patterns like the Singleton or Factory Method are powerful, but should be used when they provide a clear benefit.
7. Keep backwards compatibility in mind
In most cases, your refactoring should not alter the external behaviour of the system. Ensure that you preserve backwards compatibility when making changes. If you’re refactoring a public API, this is especially important to avoid breaking clients who rely on your code.
Tips for effective code refactoring
1. Start with smell detection
Code “smells” are signs that your code might need refactoring. Common code smells include:
- Duplicated code: Identical or near-identical code appearing in multiple places.
- Long functions: Functions that are too long or do too many things.
- Large classes: Classes that are too big and have too many responsibilities.
- Inconsistent naming: Variables, functions, or classes with unclear or inconsistent names.
Recognising these code smells can help you identify parts of the code that may benefit from refactoring.
2. Refactor one thing at a time
Try to focus on a single aspect of the code at a time when refactoring. For instance, if you’re working on a large class, start by simplifying its method names or breaking down long functions into smaller, more manageable ones. Don’t attempt to refactor everything at once.
3. Leverage modern IDE features
Many modern IDEs come with tools that can help with refactoring, such as automated code analysis, code suggestions, and even automatic refactoring for common patterns. Take advantage of these tools to make the process smoother and faster.
4. Collaborate and seek feedback
Don’t be afraid to collaborate with your team when refactoring code. Sometimes, another developer might offer a perspective or a solution that improves your approach. Conducting pair programming or code reviews can also help catch potential issues early and ensure the refactor maintains code quality.
5. Know when to stop
While refactoring can improve your code, it’s important to know when to stop. Spending excessive time refactoring code can be counterproductive, and sometimes, it’s better to focus on other aspects of the project. Be mindful of the costs involved and ensure that the benefits outweigh the time spent.
Code refactoring is an essential skill for any software developer. By following best practices such as refactoring in small increments, writing tests, and aiming for simplicity, you can maintain a high-quality codebase.
The key is to make refactoring an ongoing process and ensure that you’re constantly improving the structure of your code without compromising its functionality. When done correctly, refactoring not only makes your code more maintainable but also improves the overall development process, making your software more adaptable to future changes.
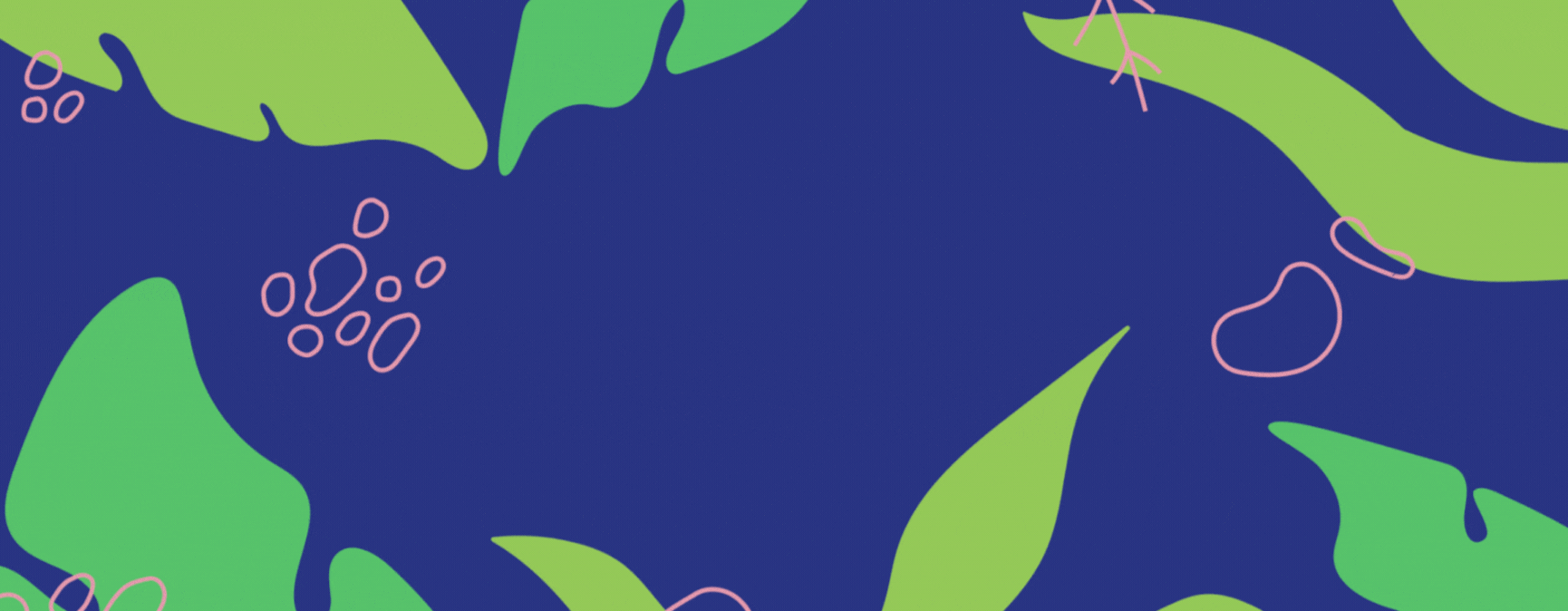